Mastering the Vercel AI SDK: A Developer's Handbook
Introduction
The Vercel AI SDK, developed by the creators of Next.js, is an open-source TypeScript toolkit designed to simplify the integration of artificial intelligence into web applications. It provides a unified API for interacting with large language models (LLMs) from providers like OpenAI, Hugging Face, and Azure OpenAI, enabling developers to build AI-powered features such as chatbots, generative content tools, and semantic search functionalities. The SDK supports popular frameworks including React, Next.js, Vue, Svelte, and Node.js, making it versatile for both front-end and back-end development. Its interoperability, streaming capabilities, and open-source nature make it a go-to choice for developers looking to create innovative AI-driven applications.
This comprehensive guide is tailored for developers of all skill levels, offering step-by-step instructions to set up, use, and extend the Vercel AI SDK. It covers installation, core features, framework integrations, advanced topics, best practices, and troubleshooting, ensuring you can build robust AI applications efficiently. Whether you're a beginner or an experienced developer, this handbook will equip you with the knowledge to leverage the SDK’s full potential.
Background and Context
The Vercel AI SDK was introduced to address the complexity of integrating LLMs into applications, which often involves provider-specific APIs and technical challenges like streaming data or error handling. Launched in 2023, the SDK has evolved through versions like 3.2 and 3.3, with features like embeddings and telemetry added to support advanced use cases such as retrieval-augmented generation (RAG) and performance monitoring (Vercel AI SDK 3.3). Its open-source nature, hosted on GitHub (Vercel AI SDK GitHub), encourages community contributions, ensuring rapid updates and feature enhancements.
The SDK’s design emphasizes interoperability, allowing developers to switch between AI providers without altering their codebase. This is particularly valuable given the diverse capabilities of providers like OpenAI (known for GPT models), Hugging Face (offering open-source models), and others. The SDK also supports streaming, which is critical for real-time applications, and integrates seamlessly with modern web frameworks, making it a preferred tool for companies like OpenAI, Replicate, and Pinecone (AI SDK Docs).
Methodology
This guide is based on insights from official Vercel resources, including the AI SDK documentation (AI SDK Docs), blog posts (Introducing the Vercel AI SDK), and release notes (Vercel AI SDK 3.2). It incorporates practical examples from the documentation and community feedback, such as X posts praising the SDK’s ease of use and fast development cycle (AI SDK Feedback). The approach assumes a Next.js project for primary examples, given its popularity and tight integration with Vercel, but includes instructions for other frameworks to ensure broad applicability. The guide also addresses common developer pain points, such as error handling and performance optimization, based on best practices outlined in the documentation.
Detailed Steps
Step 1: Getting Started
1.1 Prerequisites
Node.js (version 16 or higher) installed.
A project using a supported framework (e.g., Next.js, React, Vue, Svelte, or Node.js).
An API key from an AI provider (e.g., OpenAI, Hugging Face).
Basic knowledge of JavaScript/TypeScript and web development.
1.2 Install the Vercel AI SDK
In your project directory, install the SDK using npm:
npm install ai
Or with yarn:
yarn add ai
1.3 Set Up Your Project
For a Next.js project, create a new app:
npx create-next-app@latest my-ai-app cd my-ai-app
Ensure dependencies are installed:
npm install
1.4 Initialize the SDK
Import the SDK in your JavaScript or TypeScript file:
import { ai } from 'ai';
Configure it with your AI provider’s credentials. For OpenAI:
const openai = ai('openai', { apiKey: process.env.OPENAI_API_KEY, });
Store your API key in a .env file for security:
OPENAI_API_KEY=your-openai-api-key
Step 2: Core Features
2.1 Streaming Responses
Streaming allows real-time data delivery, ideal for chatbots or live transcription.
Example: Stream a chat completion response:
const response = await openai.chat.completions.create({ model: 'gpt-3.5-turbo', messages: [{ role: 'user', content: 'Tell me a story.' }], stream: true, }); for await (const chunk of response) { console.log(chunk.choices[0]?.delta?.content || ''); }
2.2 Chat Interfaces
The SDK simplifies building conversational UIs with chat completion APIs.
Example: Create a simple chat interaction:
const response = await openai.chat.completions.create({ model: 'gpt-3.5-turbo', messages: [{ role: 'user', content: 'Who won the World Series in 2020?' }], }); console.log(response.choices[0]?.message?.content);
2.3 Model Interoperability
Switch between providers like OpenAI, Hugging Face, or Mistral without code changes.
Example with Hugging Face:
const huggingface = ai('huggingface', { apiKey: process.env.HUGGINGFACE_API_KEY, }); const response = await huggingface.textGeneration.generate({ inputs: 'Once upon a time', parameters: { max_new_tokens: 50 }, }); console.log(response.generated_text);
Step 3: Framework Integrations
3.1 React/Next.js
The SDK provides React hooks like useChat for managing chat state.
Example: Build a chat interface in Next.js:
// pages/index.js import { useChat } from 'ai/react'; export default function ChatPage() { const { messages, input, handleInputChange, handleSubmit } = useChat(); return ( <div className="container mx-auto p-4"> <h1 className="text-2xl font-bold mb-4">AI Chat</h1> <div className="mb-4"> {messages.map((message, index) => ( <div key={index} className="mb-2"> <strong>{message.role}:</strong> {message.content} </div> ))} </div> <input value={input} onChange={handleInputChange} placeholder="Type your message..." className="border p-2 rounded w-full" /> <button onClick={handleSubmit} className="bg-blue-500 text-white p-2 rounded mt-2" > Send </button> </div> ); }
3.2 Vue
Use Vue composables for AI interactions.
Example:
// src/components/Chat.vue <script> import { useChat } from 'ai/vue'; export default { setup() { const { messages, ask } = useChat(); return { messages, ask }; }, }; </script> <template> <div> <div v-for="message in messages" :key="message.id"> {{ message.role }}: {{ message.content }} </div> <input v-model="input" @keyup.enter="ask(input)" /> </div> </template>
3.3 Svelte
Leverage Svelte stores for state management.
Example:
// src/App.svelte <script> import { writable } from 'svelte/store'; import { ai } from 'ai'; const messages = writable([]); let input = ''; async function ask() { const response = await ai.chat.completions.create({ model: 'gpt-3.5-turbo', messages: [{ role: 'user', content: input }], }); messages.update((msgs) => [ ...msgs, { role: 'assistant', content: response.choices[0].message.content }, ]); input = ''; } </script> <div> {#each $messages as message} <div>{message.role}: {message.content}</div> {/each} <input bind:value={input} on:keyup={e => e.key === 'Enter' && ask()} /> </div>
3.4 Node.js
Integrate the SDK in server-side applications.
Example:
// server.js const express = require('express'); const { ai } = require('ai'); const app = express(); app.use(express.json()); const openai = ai('openai', { apiKey: process.env.OPENAI_API_KEY }); app.post('/ask', async (req, res) => { const { question } = req.body; const response = await openai.chat.completions.create({ model: 'gpt-3.5-turbo', messages: [{ role: 'user', content: question }], }); res.json({ answer: response.choices[0].message.content }); }); app.listen(3000, () => console.log('Server running on port 3000'));
Step 4: Advanced Topics
4.1 Building Agents
Agents are autonomous AI systems that can perform tasks using tools and memory.
Example:
import { Agent } from 'ai'; const agent = new Agent({ model: 'gpt-3.5-turbo', tools: [ { type: 'function', function: { name: 'get_current_weather', description: 'Get the current weather in a given location', parameters: { type: 'object', properties: { location: { type: 'string', description: 'The city and state, e.g. San Francisco, CA' }, }, required: ['location'], }, async execute({ location }) { // Mock weather API call return `The weather in ${location} is sunny, 72°F.`; }, }, }, ], }); const response = await agent.plan('What is the weather like today in San Francisco?'); console.log(response);
4.2 Embeddings
Embeddings enable semantic search and retrieval-augmented generation (RAG).
Example:
const embedding = await openai.embeddings.create({ model: 'text-embedding-ada-002', input: 'The food was delicious and the waiter was kind.', }); console.log(embedding.data[0].embedding);
4.3 Telemetry
Monitor AI interactions with telemetry for performance insights.
Example:
import { ai } from 'ai'; import { trace } from '@vercel/otel'; const openai = ai('openai', { apiKey: process.env.OPENAI_API_KEY, experimental_telemetry: true, }); trace('chat-function', async () => { const response = await openai.chat.completions.create({ model: 'gpt-3.5-turbo', messages: [{ role: 'user', content: 'Hello' }], }); return response; });
Step 5: Best Practices
5.1 Performance Optimization
Use streaming for large responses to reduce latency.
Cache frequently asked queries to minimize API calls and costs.
Optimize model parameters (e.g., max_tokens) to balance quality and speed.
5.2 Error Handling
Handle API errors gracefully:
try { const response = await openai.chat.completions.create({ model: 'gpt-3.5-turbo', messages: [{ role: 'user', content: 'Hello' }], }); console.log(response.choices[0].message.content); } catch (error) { console.error('Error:', error.message); }
5.3 Security
Store API keys in environment variables, not in code.
Use HTTPS for all API requests to protect data.
Implement rate limiting to prevent abuse.
5.4 Scalability
Deploy on Vercel for seamless scaling and edge performance.
Use serverless functions for handling AI requests efficiently.
Step 6: Troubleshooting
Issue
Solution
API Key Invalid
Verify your API key in the provider’s dashboard and ensure it’s correctly set in .env.
Model Not Found
Check the provider’s documentation for supported models (AI SDK Docs).
Streaming Errors
Ensure stable network connectivity and correct server configuration.
Slow Responses
Optimize model parameters or enable caching for frequent queries.
Step 7: Sample Application
Below is a sample Next.js application demonstrating a chat interface using the Vercel AI SDK.
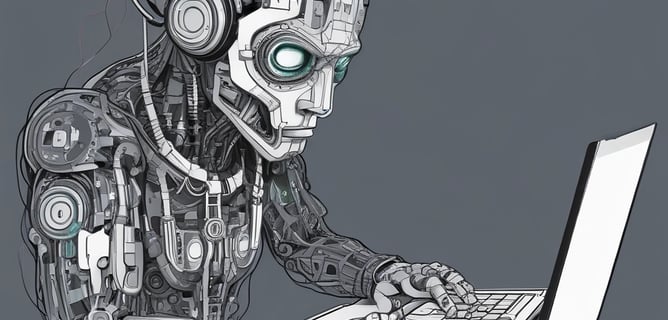
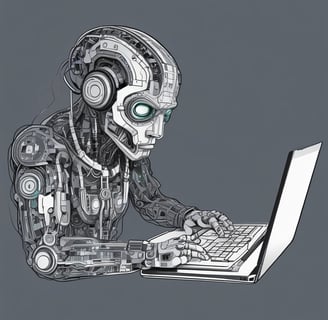